mirror of
https://github.com/kovetskiy/mark.git
synced 2025-04-23 21:32:41 +08:00
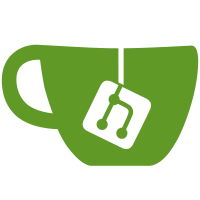
- add continue-on-error flag as a command line option - if set, doesnt exit on error and continues processing other files that were passed in - add fatalErrorHandler to handle fatal errors - if continue-on-error flag is set, does not exit - add temporary tests for continue-on-error flag - add tests in batch-tests subdirectory
36 lines
641 B
Go
36 lines
641 B
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/reconquest/pkg/log"
|
|
)
|
|
|
|
type FatalErrorHandler struct {
|
|
ContinueOnError bool
|
|
}
|
|
|
|
func NewErrorHandler(continueOnError bool) *FatalErrorHandler {
|
|
return &FatalErrorHandler{
|
|
ContinueOnError: continueOnError,
|
|
}
|
|
}
|
|
|
|
func (h *FatalErrorHandler) Handle(err error, format string, args ...interface{}) {
|
|
errorMesage := fmt.Sprintf(format, args...)
|
|
|
|
if err == nil {
|
|
if h.ContinueOnError {
|
|
log.Error(errorMesage)
|
|
return
|
|
}
|
|
log.Fatal(errorMesage)
|
|
}
|
|
|
|
if h.ContinueOnError {
|
|
log.Errorf(err, errorMesage)
|
|
return
|
|
}
|
|
log.Fatalf(err, errorMesage)
|
|
}
|